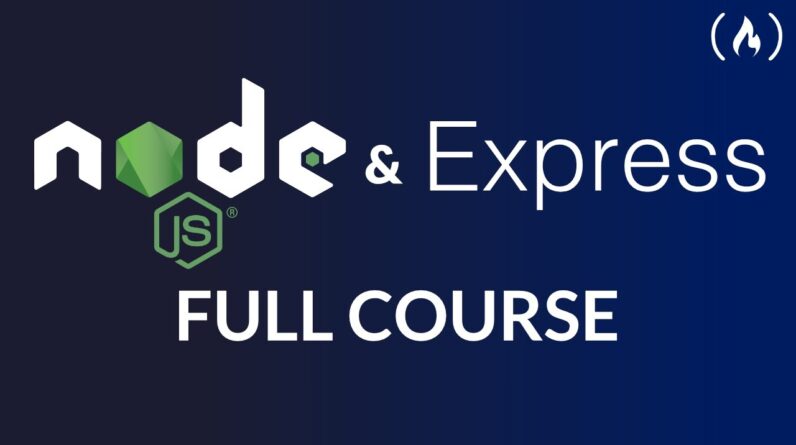
Learn how to use Node and Express in this comprehensive course. First, you will learn the fundamentals of Node and Express. Then, you will learn to build a complex Rest API. Finally, you will build a MERN app and other Node projects.
βοΈ Course developed by John Smilga. Check out his channel: https://www.youtube.com/channel/UCMZFwxv5l-XtKi693qMJptA
π» Code: https://github.com/john-smilga/node-express-course
βοΈ Course Contents βοΈ
β¨οΈ (00:00β) Introduction
β¨οΈ (01:41β) What Is Node
β¨οΈ (02:56β) Course Requirements
β¨οΈ (04:16β) Course Structure
β¨οΈ (04:59β) Browser Vs Server
β¨οΈ (07:50β) Install Node
β¨οΈ (11:08β) Repl
β¨οΈ (13:27β) Cli
β¨οΈ (19:07β) Source Code
β¨οΈ (20:27β) Globals
β¨οΈ (29:34β) Modules Setup
β¨οΈ (32:46β) First Module
β¨οΈ (45:32β) Alternative Syntax
β¨οΈ (49:50β) Mind Grenade
β¨οΈ (53:47β) Built-In Module Intro
β¨οΈ (56:31β) Os Module
β¨οΈ (1:04:13β) Path Module
β¨οΈ (1:10:06β) Fs Module (Sync)
β¨οΈ (1:18:28β) Fs Module (Async)
β¨οΈ (1:27:32β) Sync Vs Async
β¨οΈ (1:34:29β) Http Intro
β¨οΈ (1:35:58β) Http Module (Setup)
β¨οΈ (1:40:53β) Http Module (More Features)
β¨οΈ (1:45:57β) NPM Info
β¨οΈ (1:50:19β) NPM Command
β¨οΈ (1:53:10β) First Package
β¨οΈ (2:02:52β) Share Code
β¨οΈ (2:09:04β) Nodemon
β¨οΈ (2:15:04β) Uninstall
β¨οΈ (2:17:53β) Global Install
β¨οΈ (2:23:22β) Package-Lock.Json
β¨οΈ (2:25:56β) Important Topics Intro
β¨οΈ (2:27:38β) Event Loop
β¨οΈ (2:30:47β) Event Loop Slides
β¨οΈ (2:37:46β) Event Loop Code Examples
β¨οΈ (2:47:07β) Async Patterns β Blocking Code
β¨οΈ (2:54:49β) Async Patterns β Setup Promises
β¨οΈ (3:00:35β) Async Patterns β Refactor To Async
β¨οΈ (3:06:05β) Async Patterns β Nodeβs Native Option
β¨οΈ (3:12:41β) Events Info
β¨οΈ (3:14:44β) Events Emitter β Code Example
β¨οΈ (3:18:37β) Events Emitter β Additional Info
β¨οΈ (3:21:44β) Events Emitter β Http Module Example
β¨οΈ (3:25:10β) Streams Intro
β¨οΈ (3:26:18β) Streams β Read File
β¨οΈ (3:33:01β) Streams β Additional Info
β¨οΈ (3:35:05β) Streams β Http Example
β¨οΈ (3:40:29β) End Of Node Tutorial Module
β¨οΈ (3:40:46β) HTTP Request/Response Cycle
β¨οΈ (3:44:49β) Http Messages
β¨οΈ (3:55:52β) Starter Project Install
β¨οΈ (3:57:59β) Starter Overview
β¨οΈ (4:03:25β) Http Basics
β¨οΈ (4:15:09β) Http β Headers
β¨οΈ (4:24:50β) Http β Request Object
β¨οΈ (4:32:00β) Http β Html File
β¨οΈ (4:37:20β) Http β App Example
β¨οΈ (4:48:02β) Express Info
β¨οΈ (4:51:50β) Express Basics
β¨οΈ (5:03:05β) Express β App Example
β¨οΈ (5:14:31β) Express β All Static
β¨οΈ (5:18:13β) API Vs SSR
β¨οΈ (5:24:07β) JSON Basics
β¨οΈ (5:32:40β) Params, Query String β Setup
β¨οΈ (5:39:13β) Route Params
β¨οΈ (5:48:25β) Params β Extra Info
β¨οΈ (5:50:42β) Query String
β¨οΈ (6:07:31β) Additional Params And Query String Info
β¨οΈ (6:10:46β) Middleware β Setup
β¨οΈ (6:21:27β) APP.USE
β¨οΈ (6:28:31β) Multiple Middleware Functions
β¨οΈ (6:36:36β) Additional Middleware Info
β¨οΈ (6:43:26β) Methods β GET
β¨οΈ (6:49:01β) Methods β POST
β¨οΈ (6:52:53β) Methods β POST (Form Example)
β¨οΈ (7:05:31β) Methods β POST (Javascript Example)
β¨οΈ (7:21:22β) Install Postman
β¨οΈ (7:30:19β) Methods β PUT
β¨οΈ (7:41:43β) Methods β DELETE
β¨οΈ (7:50:05β) Express Router β Setup
β¨οΈ (8:05:36) Express Router β Controllers
Check out free Postman programs for students and educators to help you learn more about APIs: https://www.postman.com/company/student-program/
Learn to code for free and get a developer job: https://www.freecodecamp.org
Read hundreds of articles on programming: https://freecodecamp.org/news